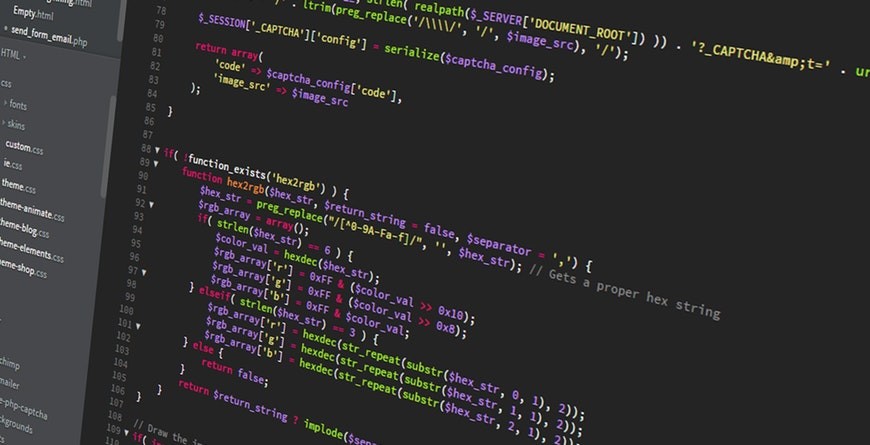
Template Method Design Pattern in Java
Template Method is a behavioral design pattern and it’s used to create a method stub and deferring some of the steps of implementation to the subclasses. Template method defines the steps to execute an algorithm and it can provide default implementation that might be common for all or some of the subclasses.
Template means Preset format like HTML templates which has fixed preset format.Similarly in template method pattern,we have a preset structure method called template method which consists of steps.This steps can be abstract method which will be implemented by its subclasses.
In Template pattern, an abstract class exposes defined way(s)/template(s) to execute its methods. Its subclasses can override the method implementation as per need but the invocation is to be in the same way as defined by an abstract class. This pattern comes under behavior pattern category.
When to use it:
- When you have a preset format or steps for algorithm but implementation of steps may vary.
- When you want to avoid code duplication,implementing common code in base class and variation in subclass.
Example Java code:
Below class contains template method called “parseDataAndGenerateOutput” which consists of steps for reading data,processing data and writing to csv file.
1.DataParser.java
view plainprint?
package org.arpit.javapostsforlearning;
abstract public class DataParser {
//Template method
//This method defines a generic structure for parsing data
public void parseDataAndGenerateOutput()
{
readData();
processData();
writeData();
}
//This methods will be implemented by its subclass
abstract void readData();
abstract void processData();
//We have to write output in a CSV file so this step will be same for all subclasses
public void writeData()
{
System.out.println(“Output generated,writing to CSV”);
}
}
In below class,CSV specific steps are implement in this class
2.CSVDataParser.java
package org.arpit.javapostsforlearning;
public class CSVDataParser extends DataParser {
void readData() {
System.out.println(“Reading data from csv file”);
}
void processData() {
System.out.println(“Looping through loaded csv file”);
}
}
In below class,database specific steps are implement in this class
3.DatabaseDataParser.java
package org.arpit.javapostsforlearning;
public class DatabaseDataParser extends DataParser {
void readData() {
System.out.println(“Reading data from database”);
}
void processData() {
System.out.println(“Looping through datasets”);
}
}
4.TemplateMethodMain.java
package org.arpit.javapostsforlearning;
public class TemplateMethodMain {
/**
* @author arpit mandliya
*/
public static void main(String[] args) {
CSVDataParser csvDataParser=new CSVDataParser();
csvDataParser.parseDataAndGenerateOutput();
System.out.println(“**********************”);
DatabaseDataParser databaseDataParser=new DatabaseDataParser();
databaseDataParser.parseDataAndGenerateOutput();
}
}
output:
Reading data from csv file
Looping through loaded csv file
Output generated,writing to CSV
**********************
Reading data from database
Looping through datasets
Output generated,writing to CSV
Template Method Class Diagram
Template Method Pattern in JDK
- All non-abstract methods of java.io.InputStream, java.io.OutputStream, java.io.Reader and java.io.Writer.
- All non-abstract methods of java.util.AbstractList, java.util.AbstractSet and java.util.AbstractMap
Important points about template method pattern:
- Template method in super class follows “the Hollywood principle”: “Don’t call us, we’ll call you”. This refers to the fact that instead of calling the methods from base class in the subclasses, the methods from subclass are called in the template method from superclass.
- Template method in super class should not be overriden so make it final
- Customization hooks:Methods containing a default implementation that may be overidden in other classes are called hooks methods. Hook methods are intended to be overridden, concrete methods are not.So in this pattern,we can provide hooks methods.The problem is sometimes it becomes very hard to differentiate between hook methods and concrete methods.
- Template methods are technique for code reuse because with this,you can figure out common behavior and defer specific behavior to subclasses.
Tag:behavioural design pattern, behavioural template method, Design method pattern, Design Pattern, java j2ee online Training, java j2ee Training and Placement by mindsmapped, java training, java Training and Placement by mindsmapped, online java j2ee training, online java training, Template method, template method design pattern, Template Method Design Pattern in Java