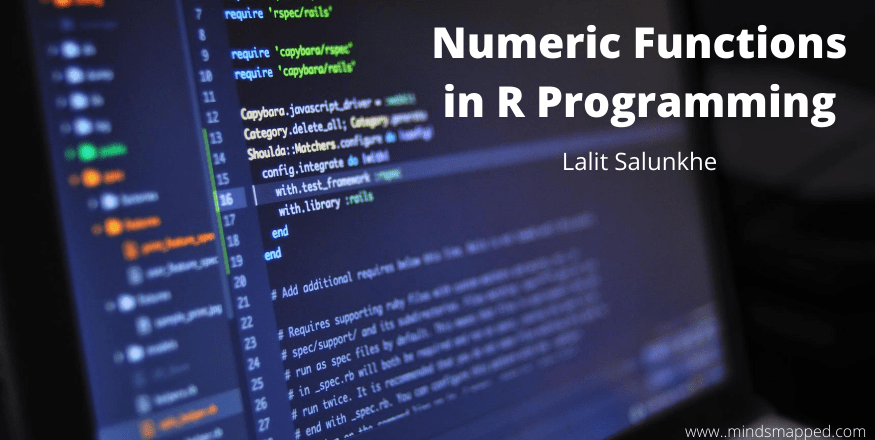
Numeric Functions in R Programming
Introduction to Numeric Functions in R Programming
The most widely used data type under R is a numeric data type. Most of the complex analysis techniques from data science are based on the numeric data type. Having said that, it becomes more important to check out the functions that will deal with this widely used data type. There are certain numeric functions in R that can be used to deal with numbers in a better way under R. In this article we are going to discuss some of the numeric functions in detail with a hand full of examples.
What Does a Numeric Function Mean?
A function that either takes a set of numeric values or a numeric vector as an input argument to perform certain tasks is considered as a numeric function in R. Following are some of the common numeric functions that we always use under R Programming. Following is the list of all numeric functions we are about to discuss through this article.
- R Programming is.numeric() Function
- R Programming abs() Function
- R Programming sqrt() Function
- R Programming ceiling() Function
- R Programming floor() Function
- R Programming round() Function
- R Programming trunc() Function
- R Programming log() Function
- R Programming log10() Function
- R Programming exp() Function
Well, the above list does not contain all of the numeric functions in R. However, we have tried to cover the most common and widely used numeric functions in R programming through this article. Let us discuss each one of them in detail with examples.
R Programming is.numeric() Function
In R Programming language, when you need to check whether the given value is a number or not, you can use the is.numeric() function which allows you to check the same. This function takes either a single element or a vector of elements as an input, and returns a logical output as TRUE if the elements are number/numeric else it returns the output as FALSE.
Let us see some examples for is.numeric() function in R. See the code below:
> # Examples for is.numeric() Function
> x <- 10
> is.numeric(x) #Checking single numeric value
[1] TRUE
> y <- c(12, 18, 24, 30)
> is.numeric(y) #Checking vector of numeric values
[1] TRUE
> z <- c(10, "Hello", 2+3i)
> is.numeric(z) #Checking Mixed values with text and complex
[1] FALSE
Here, in the first two examples, the working of is.numeric() is pretty straight forward. The single-valued number and a vector of numbers are both generating output as TRUE when we apply the is.numeric() function. However, in the third example, we have a vector of mixed data type (we have elements of numeric, string, and complex data type). Therefore, when we apply the is.numeric() function on vector z, the output is FALSE. Because the given vector is not numeric (Instead it has been coerced to string/character vector).
R Programming abs() Function
In mathematics when we need to get an absolute value for a number, we use the mod(||). In the same way, when we are working in R, we have the abs() function that returns the absolute value for the number provided as an input. This function takes a number or a vector with numeric elements as an input and returns the absolute value for each element present in the given vector.
Let’s see an example for a better realization of the abs() function.
> #Example for the abs() function
> abs(-10)
[1] 10
> p <- c(-12, -14, -16, -18)
> abs(p)
[1] 12 14 16 18
This example specifies the use of abs() function in R.
R Programming sqrt() Function
In R, whenever we need to find out the square root of a positive real number, we can use the sqrt() function. This function either can take a positive numeric value (integer or float) or a numeric vector of positive values as an input and returns the square root for the same. This function only works on positive values. If you provide a negative number as an input, we will get the NaN as an output. This means not a number. See an example below for a better understanding.
> #Example of sqrt() function
> p <- sqrt(12.28)
> print(p)
[1] 3.504283
> q <- c(18.21, 13.95, 24.78, 33,12)
> sqrt(q)
[1] 4.267318 3.734970 4.977951 5.744563 3.464102
> r <- sqrt(-23.22)
Warning message:
In sqrt(-23.22) : NaNs produced
> print(r)
[1] NaN
In this example, if you see when we provide a negative number as an argument to the sqrt() function, it returns NaN as an output.
R Programming ceiling() Function
In R, when we need to truncate the given float value to a nearest integer, we use the ceiling() function. This function in R programming takes a float (real) number or a vector of floats as an argument and then returns the smallest integer which is greater than the given number. Ideally, it rounds the given number to the smallest integer value larger than the given number. The most interesting part about the function is, it also works with the negative real numbers.
The following piece of code will show you how the ceiling() function works.
> #Examples for the ceiling() function in R
> ex_1 <- ceiling(23.48)
> print(ex_1)
[1] 24
> ex_2 <- ceiling(-18.25)
> print(ex_2)
[1] -18
> ex_3 <- ceiling(c(86.36349, 88.60154, 103.26688, 87.14673, 102.21277))
> print(ex_3)
[1] 87 89 104 88 103
- In the first example, integers greater than the given number 23.48 are 24, 25, 26, … out of which, 24 is the smallest and hence it is the output.
- In the second example, -18, -17, -16, … are the integers greater than the given number. Out of those, -18 is the smallest (remember values closer to zero are considered as larger than those far from zero while working with negative numbers) and hence it is the output when we apply the ceiling() function on given number.
- In this code, if you see the third example, the function works on each element of the vector provided and rounds it to the smallest number that is greater than the given number. Ideally, this function rounds up the value of a given floating-point number.
R Programming floor() Function
In exact opposite to the ceiling() function, the floor() function in R works. The floor() function in R takes a floating-point number or a vector consisting of the same as an argument and returns the largest integer that is smaller than the given value or set of values. When applied on a vector, it returns the largest integer value smaller than the given value for each element of the vector. In other words, we can say that the floor() function helps us in rounding down the given float to an integer value.
Example for the floor() function is as shown below:
> #Examples for the floor() function in R
> ex_1 <- floor(23.48)
> print(ex_1)
[1] 23
> ex_2 <- floor(-18.25)
> print(ex_2)
[1] -19
> ex_3 <- floor(c(86.36349, 88.60154, 103.26688, 87.14673, 102.21277))
> print(ex_3)
[1] 86 88 103 87 102
- If you see in the first example, the largest integer which is smaller than 23.48 is 23 and that’s the output after we apply the function.
- The same is the case with negative numbers. -19 is the largest integer that is smaller than -18.25 (remember, values closer to the zero are considered as larger in case of negative numbers).
- And in the third example, each element of the vector is rounded down when the function is applied.
R Programming round() Function
As far as, we have seen how to round up (ceiling() function) and round down (floor() function) any given real number. However, we haven’t seen any function that rounds the number up or down to an integer value based on the thumb rule (like if the decimal part is greater than 0.5 then round up and round down if less than 0.5). Neither we have seen any function that rounds a number up to a given number of decimal places.
All this has been taken care of into the round() function in R. This function takes a floating-point number as an argument and then rounds it off to an integer. Moreover, it also allows you to round a floating-point number up-to certain decimal places if you use “digits =” argument within the function.
Let us go towards the code section and see some examples for the round() function in R.
> #Examples for the round() function in R
> ex_1 <- round(23.55)
> print(ex_1)
[1] 24
> ex_2 <- round(-18.25)
> print(ex_2)
[1] -18
> ex_3 <- round(c(86.36349, 88.60154, 103.26688, 87.14673, 102.21277), digits = 2)
> print(ex_3)
[1] 86.36 88.60 103.27 87.15 102.21
- In the first example, the value 23.55 is rounded off to 24 since the decimal part is greater than 0.5. The round() function here works as the ceiling() function.
- In the second example, the value -18.25 is rounded off to -18 since the decimal part is lesser than 0.5. The round() function here works as the floor() function.
- In the third example, we have specified a separate argument “digits = 2“. This allows us to round the decimals up to two places as can be seen in the output.
R Programming trunc() Function
Whenever we want to truncate the decimal part from a floating-point number and just retain the integer part, we will use the trunc() function. This function takes a float or a vector of floats as an argument and truncates the decimal part out of it to just return the integer. It doesn’t round up, round down, or round off. It simply truncates the decimal part from the given float.
Example for the trunc() function can be seen below:
> #Examples for the trunc() function in R
> ex_1 <- trunc(23.55)
> print(ex_1)
[1] 23
> ex_2 <- trunc(-18.25)
> print(ex_2)
[1] -18
> ex_3 <- trunc(c(86.36349, 88.60154, 103.26688, 87.14673, 102.21277))
> print(ex_3)
[1] 86 88 103 87 102
In each example above, you can see there is no rounding up or down just truncation of the decimal/fraction part of the number. That is what the trunc() function does.
R Programming log() Function
The natural logarithms are one of the important parts of our lives. The same is the case with programmers. They are every now and then in need to check for the natural logarithms while developing a program. In R, we have a log() function that allows us to get the natural logarithm for a given positive real number (numeric or integer). This function takes a single number or a vector of numbers as an input argument to return the natural logarithm.
Let us go through an example for a better realization.
> #Examples for the log() function in R
> ex_1 <- log(2) #Integer
> print(ex_1)
[1] 0.6931472
> ex_2 <- log(8.243) #Floating-point
> print(ex_2)
[1] 2.109364
> ex_3 <- log(c(86.36349, 88.60154, 103.26688, 87.14673, 102.21277)) #Vector
> print(ex_3)
[1] 4.458565 4.484149 4.637317 4.467593 4.627057
In the example above, we can see the function works perfectly with the real values and a vector consisting of the real values. However, there are some other properties of the log() function in R those are listed below:
- The log() function doesn’t work with the negative values. If you try applying this function on negative value (numeric/integer), it returns NaN. Full form or meaning of the NaN is “Not a Number“.
- The log() function if applied on the NaN, returns a NaN.
- -Inf and Inf are the two different data types. meaning negative infinite and positive infinite respectively. log() function applied on -Inf returns the NaN; whereas if applied on Inf, it returns Inf itself as an output.
- log() function applied on zero, returns the negative infinite (-Inf) as an output.
- The natural logarithm is also called as a logarithm at a base n (stands for a natural number).
See the code below for a better realization.
#log() for a negative number
> log(-1)
[1] NaN
Warning message:
In log(-1) : NaNs produced
#log() of NaN
> log(NaN)
[1] NaN
#log() of zero
> log(0)
[1] -Inf
#log() of -Inf
> log(-Inf)
[1] NaN
Warning message:
In log(-Inf) : NaNs produced
#log() of Inf
> log(Inf)
[1] Inf
R Programming log10() Function
When we need to get the natural logarithm or log to the base n, we use the log() function in R. On the similar lines when we need to get the logarithm at the base 10, we have a function called log10() in R. This function takes a positive real number or a vector of positive real number as an input and returns the log value for the same at base 10.
Let us go through some examples that give us an idea about how the log10() function works in R.
> #Examples for the log10() function in R
> ex_1 <- log10(2)
> print(ex_1)
[1] 0.30103
> ex_2 <- log10(8.243)
> print(ex_2)
[1] 0.9160853
> ex_3 <- log10(c(86.36349, 88.60154, 103.26688, 87.14673, 102.21277))
> print(ex_3)
[1] 1.936330 1.947441 2.013961 1.940251 2.009505
The same way we have discussed in the log() function, we have same properties for the log10() function when it comes to deal with the negative values, NaNs, -Inf, Inf, and zero. See an example code for the same.
#log10() for zero
> log10(0)
[1] -Inf
#log10() for -Inf
> log10(-Inf)
[1] NaN
Warning message:
NaNs produced
#log10() for Inf
> log10(Inf)
[1] Inf
#log10() for NaN
> log10(NaN)
[1] NaN
#log10() for negative number
> log10(-0.98)
[1] NaN
Warning message:
NaNs produced
R Programming exp() Function
In mathematics, we have an exponential constant (“e”) which has an approximate value as 2.71828… In R programming, function exp() is working as this exponential constant. This function takes a numeric value as an argument and raises the power of this mathematical exponential constant (2.71828…) up to that numeric value and returns the output. This function works with both negative and positive numeric values.
The code below will help us to get a better idea of the working of exp() function in R programming.
> #Examples for the exp() function in R
> ex_1 <- exp(2)
> print(ex_1)
[1] 7.389056
> ex_2 <- exp(-8.243)
> print(ex_2)
[1] 0.0002630938
> ex_3 <- exp(c(86.36349, 88.60154, 103.26688, 87.14673, 102.21277))
> print(ex_3)
[1] 3.215046e+37 3.014116e+38 7.050763e+44 7.036294e+37 2.457208e+44
Interestingly, when we use the exp() function over a vector, it considers each element of the vector separately and then applies the function to raise the power of exponential constant by the value provided.
Summary
In this article, we tried to deal with some widely as well as commonly used built-in numeric functions in R. These are not the only numeric functions present in R; you can find more built-in numeric functions, however these are the most commonly used ones. Let’s stop this article here and in the next article we will come up to you with some widely used character Functions present in R. Until then stay home, stay safe! 🙂
Tag:abs() function, built-in numeric functions in R, built-in numeric functions in R programming, ceiling() function, floor() function, is.numeric() function, log() function, log10() function, Numeric Functions in R, Numeric Functions in R programming, R numeric functions, round() function, sqrt() function, trunc() function, what is numeric functions