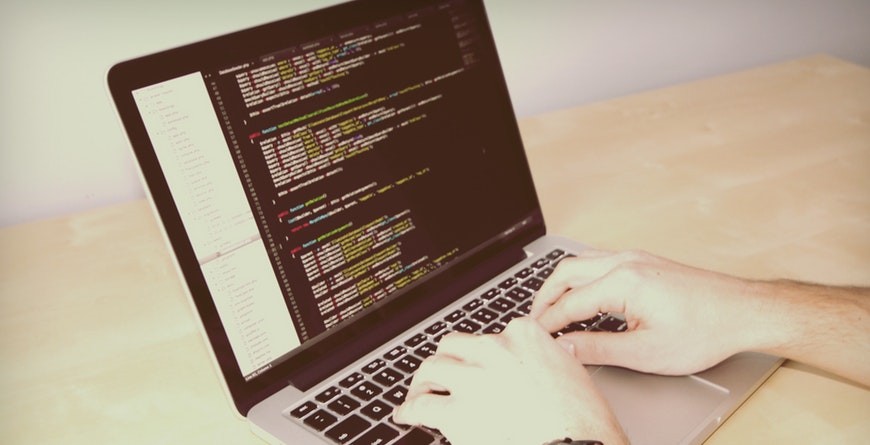
Listeners: Can Java Hear You?
What Sort of Listener Are You?
If we tried to implement a full graphical interface in a program by using MouseListener, we’d have a lot of work to do. We’d have to draw all the buttons using drawing instructions in our program, then wait for mouse clicks. Once we got a click on something we’d have to figure out what had been clicked on by the location of the click in our window. Then we’d have to redraw the button (or slider, or whatever) to show that it had been clicked. Then we’d have to continue responding to the mouse by figuring out what the user is doing (clicking and releasing, clicking and dragging, or what) and make our program and the picture on the screen respond appropriately.
What is a Listener?
A Listener is a connection between your program and something that creates an Event. An Event is a communication from a device on the computer or a program to something outside itself on the computer, like another program or device (through a program associated with that device, like its device driver.) In the case of our mouse program, the Listener is the connection between the mouse’s button and our program. Whenever the left button is clicked, an Event is generated that contains what happened (a left-click) and the location in our window where it happened.
If the click happens outside our program’s window, an Event is generated, but our program isn’t included in the recipients of the message. If our program doesn’t have a Listener, the mouse click Event gets passed to our program but is ignored.
Introduction to Event Listeners
If you have read any of the component how-to pages, you probably already know the basics of event listeners.
Let us look at one of the simplest event handling examples possible. It is called Beeper, and it features a button that beeps when you click it.
Click the Launch button to run Beeper using Java™ Web Start (download JDK 7 or later). Alternatively, to compile and run the example yourself, consult the example index.
You can find the entire program in Beeper.java. Here is the code that implements the event handling for the button:
public class Beeper … implements ActionListener {
…
//where initialization occurs:
button.addActionListener(this);
…
public void actionPerformed(ActionEvent e) {
…//Make a beep sound…
}
}
The Beeper class implements the ActionListener interface, which contains one method: actionPerformed. Since Beeper implements ActionListener, a Beeper object can register as a listener for the action events that buttons fire. Once the Beeper has been registered using the Button addActionListener method, the Beeper’s actionPerformedmethod is called every time the button is clicked.
Concepts: Low-Level Events and Semantic Events
Events can be divided into two groups: low-level events and semantic events. Low-level events represent window-system occurrences or low-level input. Everything else is a semantic event.
Examples of low-level events include mouse and key events both of which result directly from user input. Examples of semantic events include action and item events. A semantic event might be triggered by user input; for example, a button customarily fires an action event when the user clicks it, and a text field fires an action event when the user presses Enter. However, some semantic events are not triggered by low-level events, at all. For example, a table-model event might be fired when a table model receives new data from a database.
Tag:Event listeners, Event Listeners Introduction, Introduction to event listeners, java j2ee online Training, java j2ee Training and Placement by mindsmapped, java training, java Training and Placement by mindsmapped, Listeners, Listeners: Can Java Hear You?, low level events, online java j2ee training, online java training, semantic events, what is listeners