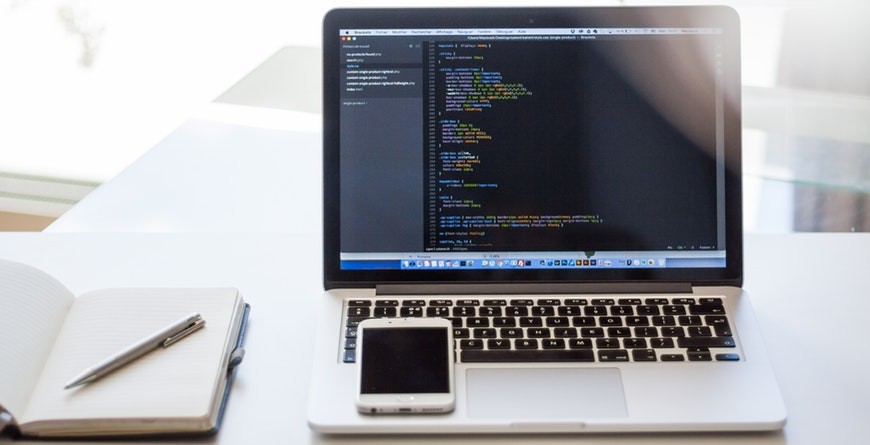
Jackson example – Read and write JSON
Java JSON Processing API is not very user-friendly and doesn’t provide features for automatic transformation from JSON to Java object and vice versa. Luckily we have some alternative APIs that we can use for JSON processing. In the last article, we learned about Google Gson API and saw how easy to use it.
Jackson project has implemented a very useful Streaming API which is also called incremental mode. This is the most efficient way to process JSON content. It has the lowest memory and processing overhead, and can often match the performance of many binary data formats available on Java platform. It’s a bit tricky to use though because you have to handle JSON data in all it’s details.
1. Create a JSON representation and write it to a File
JacksonStreamAPIExample.java:
package com.javacodegeeks.java.core;
import java.io.File;
import java.io.IOException;
import org.codehaus.jackson.JsonEncoding;
import org.codehaus.jackson.JsonFactory;
import org.codehaus.jackson.JsonGenerationException;
import org.codehaus.jackson.JsonGenerator;
import org.codehaus.jackson.map.JsonMappingException;
public class JacksonStreamAPIExample {
private static final String jsonFilePath = “C:\\Users\\nikos7\\Desktop\\filesForExamples\\jsonFile.json”;
public static void main(String[] args) {
try {
JsonFactory jsonfactory = new JsonFactory();
File jsonFile = new File(jsonFilePath);
JsonGenerator jsonGenerator = jsonfactory.createJsonGenerator(jsonFile, JsonEncoding.UTF8);
jsonGenerator.writeStartObject();
jsonGenerator.writeStringField(“domain”, “javacodegeeks.com”);
jsonGenerator.writeNumberField(“members”, 200);
jsonGenerator.writeFieldName(“names”);
jsonGenerator.writeStartArray();
jsonGenerator.writeString(“John”);
jsonGenerator.writeString(“Jack”);
jsonGenerator.writeString(“James”);
jsonGenerator.writeEndArray();
jsonGenerator.writeEndObject();
jsonGenerator.close();
System.out.println(“The file was created successfully”);
} catch (JsonGenerationException e) {
e.printStackTrace();
} catch (JsonMappingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
jsonFile.json:
{“domain”:”javacodegeeks.com”,”members”:200,”names”:[“John”,”Jack”,”James”]}
2. Parse a JSON file
JsonParser is the jackson streaming API to read json data, we are using it to read data from the file and then parseJSON() method is used to loop through the tokens and process them to create our java object. Notice that parseJSON() method is called recursively for “address” because it’s a nested object in the json data. For parsing arrays, we are looping through the json document.
package com.javacodegeeks.java.core;
import java.io.File;
import java.io.IOException;
import org.codehaus.jackson.JsonFactory;
import org.codehaus.jackson.JsonGenerationException;
import org.codehaus.jackson.JsonParser;
import org.codehaus.jackson.JsonToken;
import org.codehaus.jackson.map.JsonMappingException;
public class JacksonStreamAPIExample {
private static final String jsonFilePath = “C:\\Users\\nikos7\\Desktop\\filesForExamples\\jsonFile.json”;
public static void main(String[] args) {
try {
JsonFactory jsonfactory = new JsonFactory();
//input file
File jsonFile = new File(jsonFilePath);
JsonParser jsonParser = jsonfactory.createJsonParser(jsonFile);
// Begin the parsing procedure
while (jsonParser.nextToken() != JsonToken.END_OBJECT) {
String token = jsonParser.getCurrentName();
if (“domain”.equals(token)) {
// get the next token which will be the value…
jsonParser.nextToken();
System.out.println(“domain : “+jsonParser.getText());
}
if (“members”.equals(token)) {
jsonParser.nextToken();
System.out.println(“members : ” + jsonParser.getIntValue());
}
if (“names”.equals(token)) {
System.out.println(“names :”);
//the next token will be ‘[‘ that means that we have an array
jsonParser.nextToken();
// parse tokens until you find ‘]’
while (jsonParser.nextToken() != JsonToken.END_ARRAY) {
System.out.println(jsonParser.getText());
}
}
}
jsonParser.close();
} catch (JsonGenerationException e) {
e.printStackTrace();
} catch (JsonMappingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
domain : javacodegeeks.com
members : 200
names :
John
Jack
James
Jackson JSON API is easy to use and provide a lot of options for the ease of developers working with JSON data. Download project from below link and play around with it to explore more options about Jackson Json API.
Tag:Jackson, Jackson example, Jackson example – Read and write JSON, java j2ee online Training, java j2ee Training and Placement by mindsmapped, java training, java Training and Placement by mindsmapped, JSON, online java j2ee training, online java training, Parse a Json File, read and write json, Read Json, write Json