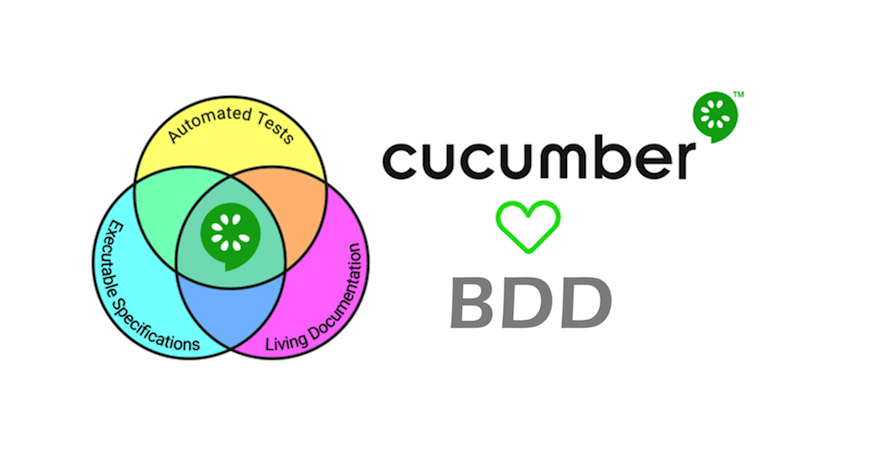
Introduction to Cucumber – Part 1
What is Cucumber?
Well, Today’s software development uses TDD and BDD practices and requires continuous integration and continuous deployment. Automation testers are needed to develop robust, clean, and thorough frameworks for regression testing, functional testing, and acceptance testing.
What is Behavior Driven Development (BDD)?
BDD (Behavior Driven development) is a combination of Business side and a technology side. It’s used to write a human readable plain text to describe a feature of an application in term of behavior in software. BDD describes the behavior of the system and its business focused or customer focused.
Why Behavior Driven Development (BDD)?
Imagine a situation where you have written a code to develop one feature and its working as expected. What if your Product Owner/BA or may be CEO of the company ask how the feature works? Or what is the test you have written for this feature? Well, you need to explain in a plain text as they can understand about a feature in Business language not by technical code. BDD follows simple plain text where anybody can understand, but it has its own syntax. There are tools which supports BDD. Tools used to convert simple text into executable code to see the behavior of the software.
For example, let’s consider developing a Login feature of any application.
Let’s describe the above Feature in steps as below:
- Go to website – Gmail
- Enter Username and Password
- Click on Sign in
- Validate whether Login is successful or not
In the above example its very clear that even anybody who is not techie can able to understand what the feature is about and how it works.
So, in the BDD the approach is similar. i.e. the coding is done exactly in the similar way.
Why Cucumber?
So far we have focused on technology side i.e. TDD (Test Driven Development) with Selenium testing. When we talk about Cucumber in BDD-Domain driven Design, it brings Business intact together. Business is much more involved in designing the application and for test apps.
Cucumber is one of the open source tools which supports BDD practice. There are other tools as well such as Jbehave, Nbehave, Specflow which supports BDD. Cucumber uses a Gherkin language which is basically a Business language, Domain specific language where anybody can understand the behavior of software as Gherkin format is in the plain text. Here the tests are written in Gherkin language. It uses Regular expressions to match Gherkin in steps. Cucumber is implemented in different programming languages such as Ruby, Java, Jruby, Groovy, Javascript, .Net, C++ PHP etc. Well, Cucumber was originally developed using Ruby programming.
In Cucumber we create a Feature file which contains of multiple User stories or scenarios and Scenarios contains steps. The coding in done in the similar manner like in the above example: – Login feature.
Cucumber reads the lines which are coded using keywords and executes the scenarios in according to the steps that are mentioned. And thus, called a Behavior driven approach.
Below are the few syntaxes used in the Gherkin:
- Feature
- Background
- Scenario
- Given
- When
- Then
- And
- But
- Scenario outline
- Examples
- Scenario templates
Example:
Let’s imagine that you are building a completely new e-commerce platform. One of the key features of any online shopping platform is the ability to buy products. But before buying anything, customers should be able to tell the system which products they are interested in buying. You need a basket.
Let’s write our first user-story:
Feature: Product basket
In order to buy products
As a customer
I need to be able to put interesting products into a basket
Before we begin to work on this feature, we must fulfil a promise of any user-story and have a real conversation with our business stakeholders. They might say that they want customers to see not only the combined price of the products in the basket, but the price reflecting both the VAT (20%) and the delivery cost (which depends on the total price of the products):
Feature: Product basket
In order to buy products
As a customer
I need to be able to put interesting products into a basket
Rules:
- VAT is 20%
– Delivery for basket under £10 is £3
– Delivery for basket over £10 is £2
So, as you can see, it already becomes tricky (ambiguous at least) to talk about this feature in terms of rules. What does it mean to add VAT? What happens when we have two products, one of which is less than £10 and another that is more? Instead you proceed with having a back-and-forth chat with stakeholders in form of actual examples of a customer adding products to the basket. After some time, you will come up with your first behavior examples (in BDD these are called scenarios):
Feature: Product basket
In order to buy products
As a customer
I need to be able to put interesting products into a basket
Rules:
- VAT is 20%
- Delivery for basket under £10 is £3
- Delivery for basket over £10 is £2
Scenario: Buying a single product under £10
Given there is a “Sith Lord Lightsaber”, which costs £5
When I add the “Sith Lord Lightsaber” to the basket
Then I should have 1 product in the basket
And the overall basket price should be £9
Scenario: Buying a single product over £10
Given there is a “Sith Lord Lightsaber”, which costs £15
When I add the “Sith Lord Lightsaber” to the basket
Then I should have 1 product in the basket
And the overall basket price should be £20
Scenario: Buying two products over £10
Given there is a “Sith Lord Lightsaber”, which costs £10
And there is a “Jedi Lightsaber”, which costs £5
When I add the “Sith Lord Lightsaber” to the basket
And I add the “Jedi Lightsaber” to the basket
Then I should have 2 products in the basket
And the overall basket price should be £20
Each scenario always follows the same basic format:
Scenario: Some description of the scenario
Given some context
When some event
Then outcome
Each part of the scenario – the context, the event, and the outcome – can be extended by adding the And or But keyword:
Scenario: Some description of the scenario
Given some context
And more context
When some event
And second event occurs
Then outcome
And another outcome
But another outcome
There’s no actual difference between, Then, And But or any of the other words that start each line. These keywords are all made available so that your scenarios are natural and readable. Thus, a feature description is divided into user scenarios which can be coded using keywords as given in the above snippets.
There is another thing in Gherkin topic called Scenario outline.
A scenario will run as a unique scenario for each line in its example table. the Scenario Outline keyword can be used to repeat the same steps with different values or arguments being passed to the step definitions. This is helpful if you want to test multiple arguments in the same scenario. For example, we have a Palindrome string example that verifies if the string being passed is a Palindrome or not:
Scenario: Valid Palindrome
Given I entered string “Refer”
When I test it for Palindrome
Then the result should be “true”
Scenario: Invalid Palindrome
Given I entered string “Coin”
When I test it for Palindrome
Then the result should be “false”
We can repeatedly do something like above and check for each word that we want to test, but that will make our hard to maintain with a lot of repetitions. Instead, we can use scenario outline to add different inputs or arguments to the same scenario. We can re-write like this:
Scenario Outline: Check if String is Palindrome
Given I entered word <wordToTest>
When I test it for Palindrome
Then the output should be <output>
Examples:
| wordToTest | output |
| "Refer" | "true" |
| "Coin" | "false" |
| "Space" | "false" |
| "racecar" | "true" |
As you can see from above, instead of keyword Scenario, we use Scenario Outline. And we use <column-name-here> inside the Given statement and Then statement to determine their values. We also add the Examples table. This is a tabular format of data that contains the values that will be passed to the Scenario. The scenario will run for each row of the Example table. For example, when Cucumber starts to run this program, first, it will use the word “Refer” to check for palindrome and the output should be “true”. Next, it will run the same scenario, but using the word “Coin” and output “false”. The scenario will run for all the rows of the table.
Below is the one more better example for Scenario Outline, with different combinations.
Feature: Amazon create a account page should have verification on all the fields.
Scenario Outline: All of the fields should display an error when not populated on form submission
Given I am on the site homepage
When I click on “Sign in link” on the “Home” page
And I click on “register” on the “sign in” page
And I enter “test@test.com” into all fields on the page
And I clear the “<filed>” field on the Create Account page
And I click on “submit” on the “Create Account” page
Then the “<error>” on the “<Create Account>” page should be “visible”
Examples:
| field | error |
| name | missing_name_error |
| email | missing_email_error |
| password | missing_password_error |
| confirm_password | missing_confirm_password_error |
Let’s have a look at the one more important topic i.e. Gherkin: Background:
Backgrounds is another convenient way to make tests little bit shorter, more readable and more maintainable. Background is generally used when all of your scenarios have exact same first step or first couple of few steps.
Let’s take another example to how Backgrounds is been used in the code for better understanding on this.
Feature: Insert detailed description here
Scenario: Examples 1
Given I sign in as “user1”
When I click on “My Account” on the “Home” page
Then I should be on the “My Account” page
Scenario: examples 2
Given I sign in as “user1”
When I click on “Log out” on the “Home” page
Then I should be on the “Log in” page
Scenario: examples 3
Given I sign in as “user1”
When I click on “My Account” on the “Home” page
And I click on “Log out” on the “My Account” page
Then I should be on the “Log in” page
In the above snippet example we can see all the Scenarios have the same first step and some have same second step as well. If all the scenarios do not have same first step, then we cannot use background in that case. What Background really does is that it executes first before all the scenarios. So as we have same first step in all the scenarios we can put them in Background like this:
Feature: Insert detailed description here
Background: Insert background description here
Given I sign in as “user1”
Scenario: Examples 1
Given I sign in as “user1”
When I click on “My Account” on the “Home” page
Then I should be on the “My Account” page
Scenario: examples 2
Given I sign in as “user1”
When I click on “Log out” on the “Home” page
Then I should be on the “Log in” page
Scenario: examples 3
Given I sign in as “user1”
When I click on “My Account” on the “Home” page
And I click on “Log out” on the “My Account” page
Then I should be on the “Log in” page
So, it executes background first then executes scenarios.
Next we have another topic to cover as whole is that Step Definition: whenever we write Step Definition, the pattern Given, When, then does not matter. Basically, they are ignored here as far the code goes. Because the regular expression starts after Given, When and Then and ends at the end of line
Let’s take one example for this:
When I click on “My Account” on the “Home” page
When /^I click on “(. *)” on the “(. *)” page$/ do |element, page|
$page_loader.load(page).get_element(element).click
end
In the above example, the top is the step and the bottom is the step definition. In the second line we have parenthesis in the quotes defines a parameter. Here the parameters are “My Account” and “Home”. We are passing these two parameters in the page element and doing a click action. This is simple Page object model where we are finding the page element and clicking on it.
So, in the Step definition we cannot have multiple things to find the match. Every time we execute something which matches this, it must execute the same code. Otherwise it will be an unambiguous match. So, you can have your step definition has to match one thing. We cannot have step definition matching multiple things.
Let’s summarize about what is the difference between BDD vs traditional automation in order to understand why we need BDD practice.
BDD Approach vs Traditional Automation
BDD approach | Traditional Automation |
Its plain text and easy to understand | It is basically a full of code and hard to understand |
Its easy to follow for anybody, BA/QA/DEV/Automation Test Engineer and all will be in the same page | The code can be understandable only by Automation Test engineers or Developers |
As its in the plain text format, BDD can be shared even to Stakeholders | Not possible to share |
Easy to learn and Implement | It requires more knowledge to design and implement |
In this article we have tried to cover the importance of BDD approach and how that can be implemented using Cucumber tool. In the next article will see about how we use plain text in the Cucumber tool and generates scripts and how it is integrated in the project, what are the things we should be knowing and how we use Gherkin language in the Cucumber Framework.
Stay tuned to know more about in the next article….