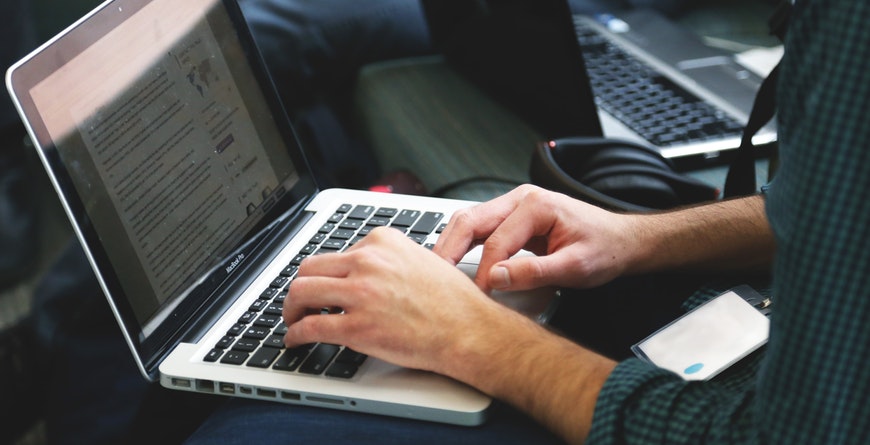
How to remove duplicates from ArrayList in java
You can remove duplicates or repeated elements from ArrayList in Java by converting ArrayList into HashSet in Java. but before doing that just keep in mind that Set doesn’t preserver insertion order which is guaranteed by List, in fact that’s the main difference between List and Set in Java. So when you convert ArrayList to HashSet all duplicates elements will be removed but insertion order will be lost. Let’s see this in action by writing a Java program to remove duplicates from Array List in Java
This example shows how to remove duplicate from ArrayList. The easiest way to remove duplicate is by passing the List to an Set. As set doesn’t support duplicates it will omit the duplicate values. Once you have the Set you can again pass it back to ArrayList.
Duplicate Values From Java ArrayList using HashSet
import java.util.ArrayList;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
public class RemoveDuplicate {
public static void main(String[] args) {
List sourceList = new ArrayList();
sourceList.add(“object1”);
sourceList.add(“object2”);
sourceList.add(“object2”);
sourceList.add(“object3”);
sourceList.add(“object4”);
sourceList.add(“object2”);
List newList = new ArrayList(new HashSet(
sourceList));
Iterator it = newList.iterator();
while (it.hasNext()) {
System.out.println(it.next());
}
}
}
Output
object3
object4
object1
object2
In this above example we have removed duplicates from the ArrayList but we will loose the sequencing index. If you want to preserve the order of data use LinkedHashSet rather HashSet. Find an example below
Duplicate Values From Java ArrayList using LinkedHashSet
import java.util.ArrayList;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.List;
public class RemoveDuplicate {
public static void main(String[] args) {
List sourceList = new ArrayList();
sourceList.add(“object1”);
sourceList.add(“object2”);
sourceList.add(“object2”);
sourceList.add(“object3”);
sourceList.add(“object4”);
sourceList.add(“object2”);
List newList = new ArrayList(new LinkedHashSet(
sourceList));
Iterator it = newList.iterator();
while (it.hasNext()) {
System.out.println(it.next());
}
}
}
Output
object1
object2
object3
object4
That’s all about how to remove duplicates from ArrayList in Java. Though there are multiple ways to do this, I think using LinkedHashSet is the simplest one because its simple and also preserve the order of elements.
Tag:Arraylist, Arraylist in JAVA, Basic Java, How to remove duplicates from ArrayList in java, java, java j2ee online Training, java j2ee Training and Placement by mindsmapped, java training, java Training and Placement by mindsmapped, online java j2ee training, online java training, remove duplicates from ArrayList in java